Both of these are used in programming, and can be used in both C# for Unity and C++ for UE4 (examples will be shown below later). First, as supported by
(Codingbat, 2015), an Array is a method used to store a collection of elements in code, such as variables, and everything inside the array will be publicly available from an index number. The index number is above the variable in the table that's used for an array, which are made out of square brackets. Each array can have an assigned variable type, such as an int or string. Here is an example of one below quoted from Coding Bat:
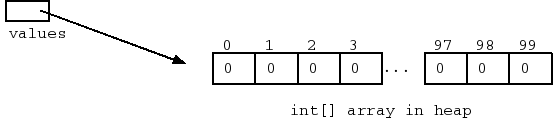 |
"In the heap, the array is a block of memory made of all the elements together. Each element in the array is identified by a zero-based index number: 0, 1, 2, ... and so on. An attempt to access an index outside the 0..length-1 range will fail with a runtime exception. When first created, all of the elements in the array are set to zero. So the memory drawing for the code above looks like:" (Codingbat, 2015) |
Next up are Loops, which can actually work hand-in-hand with Arrays (hence the post title)! As helped and posted by
(Msdn.microsoft c, 2015), the official Microsoft website for C#: "
A loop is a statement, or set of statements, that are repeated for a specified number of times or until some condition is met. The type of loop you use depends on your programming task and your personal coding preference." So it basically is a piece of code that repeats itself, sometimes for a set amount of times. It can have various practical applications in games, such as a spawn point in a platformer game that's set to create 15 enemies and then stop.
A large comparison between loops in C# and C++ is the Foreach Loop in C#, which can help repeat Arrays in code as a simple procedure. This can help save time and space in code, as rather than dedicating an entire variable in C# just for one simple array, the Foreach Loop does a large part of this on its own, making your code much more efficient. Here's an example of it below being used in C#, along with Arrays from Microsoft:
// An array of integers
int[] array1 = {0, 1, 2, 3, 4, 5};
foreach (int n in array1)
{
System.Console.WriteLine(n.ToString());
}
// An array of strings
string[] array2 = {"hello", "world"};
foreach (string s in array2)
{
System.Console.WriteLine(s);
}.
No comments:
Post a Comment